錯(cuò)誤處理往往是最容易被忽視的環(huán)節(jié),但恰恰是它決定了應(yīng)用的健壯性和用戶體驗(yàn)。分享8個(gè)實(shí)用的JavaScript錯(cuò)誤處理技巧,幫助我們構(gòu)建更可靠的應(yīng)用程序。
1. 使用 try-catch 包裝異步代碼
許多開發(fā)者認(rèn)為 try-catch 只能處理同步代碼,實(shí)際上通過適當(dāng)?shù)姆绞剑材軆?yōu)雅地處理異步操作。
// ? 錯(cuò)誤示范
async function fetchUserData() {
const response = await fetch('/api/users');
const data = await response.json();
return data;
}
// ? 正確示范
async function fetchUserData() {
try {
const response = await fetch('/api/users');
const data = await response.json();
return data;
} catch (error) {
// 區(qū)分不同類型的錯(cuò)誤
if (error instanceof TypeError) {
console.error('網(wǎng)絡(luò)請(qǐng)求失敗:', error.message);
// 可以選擇重試或返回緩存數(shù)據(jù)
} else {
console.error('解析數(shù)據(jù)失敗:', error.message);
}
// 返回默認(rèn)值或者拋出自定義錯(cuò)誤
return [];
}
}
2. 實(shí)現(xiàn)全局錯(cuò)誤處理器
全局錯(cuò)誤處理可以捕獲未被局部 try-catch 捕獲的錯(cuò)誤,是構(gòu)建可靠應(yīng)用的最后一道防線。
// 處理普通JavaScript錯(cuò)誤
window.onerror = function(message, source, lineno, colno, error) {
console.error({
message,
source,
lineno,
colno,
error: error?.stack
});
// 向錯(cuò)誤監(jiān)控服務(wù)發(fā)送報(bào)告
sendErrorReport({
type: 'js_error',
details: {message, source, lineno, colno}
});
return true; // 防止錯(cuò)誤繼續(xù)向上傳播
};
// 處理未捕獲的Promise錯(cuò)誤
window.addEventListener('unhandledrejection', function(event) {
console.error('未處理的Promise錯(cuò)誤:', event.reason);
event.preventDefault(); // 阻止默認(rèn)處理
});
3. 自定義錯(cuò)誤類型
創(chuàng)建自定義錯(cuò)誤類型可以更好地區(qū)分和處理不同類別的錯(cuò)誤。
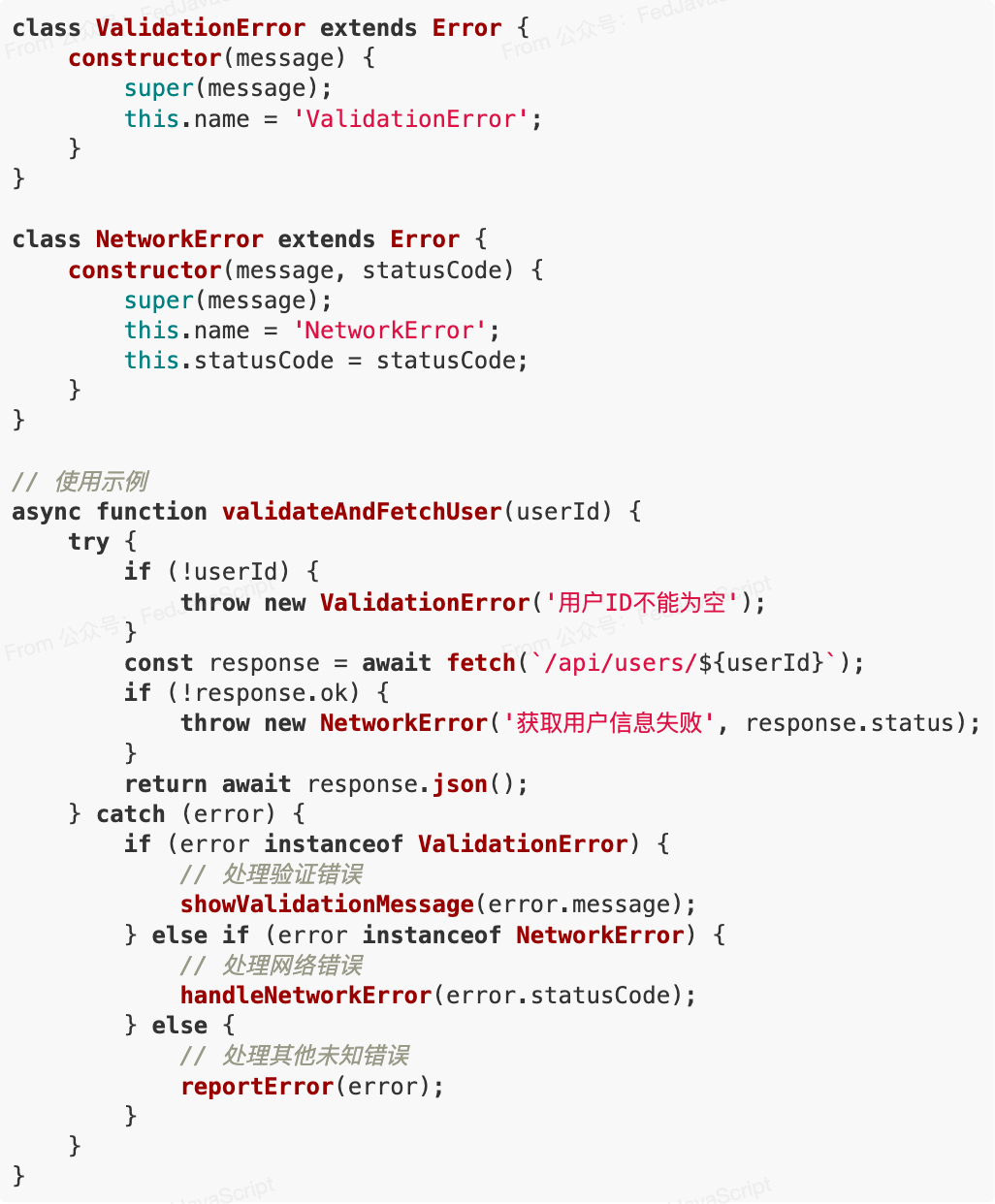
4. 優(yōu)雅的錯(cuò)誤降級(jí)處理
當(dāng)遇到錯(cuò)誤時(shí),應(yīng)該提供合理的降級(jí)方案,而不是讓應(yīng)用直接崩潰。
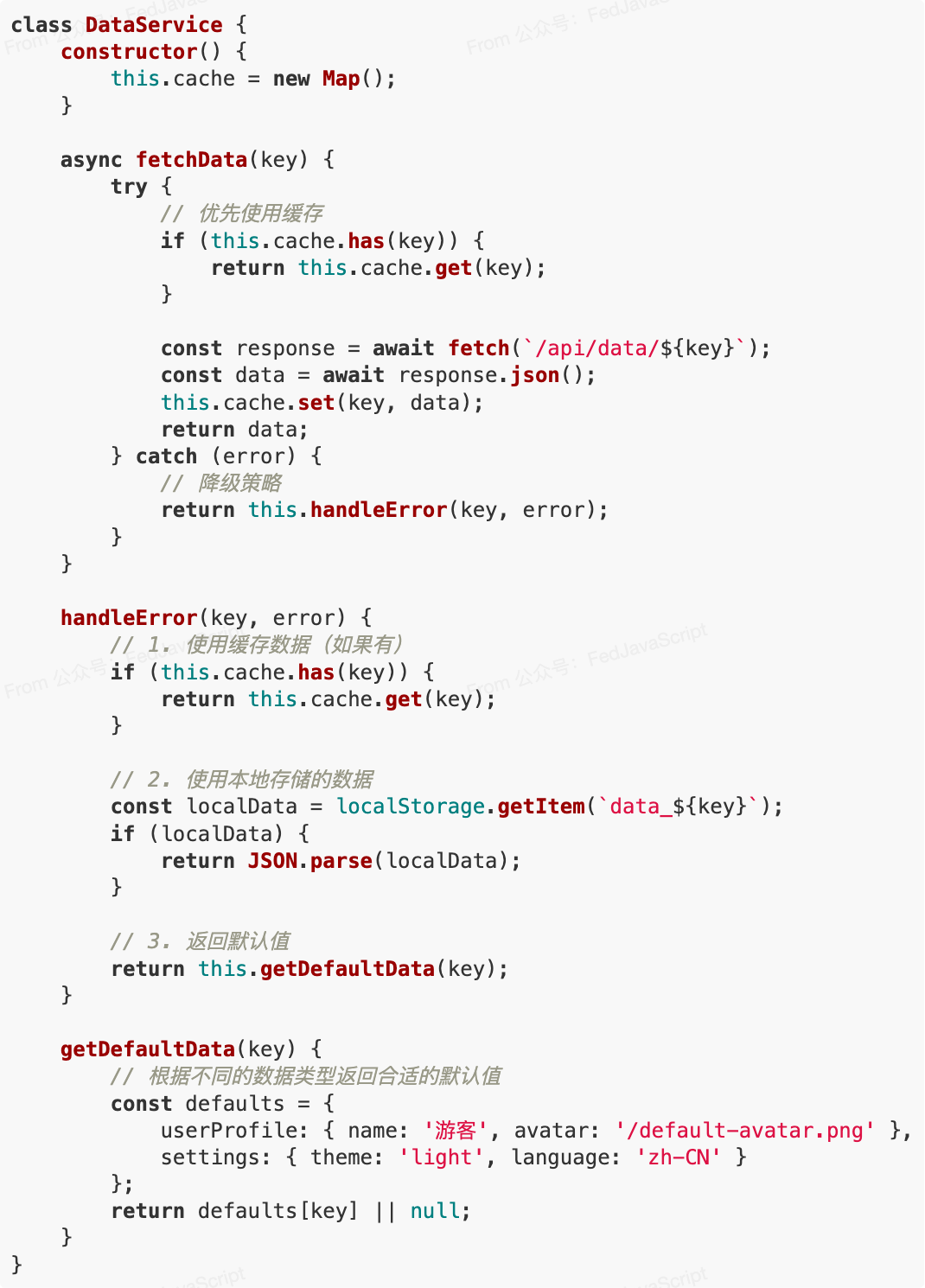
5. 錯(cuò)誤邊界處理
在React應(yīng)用中,使用錯(cuò)誤邊界可以防止整個(gè)應(yīng)用因局部錯(cuò)誤而崩潰。雖然普通JavaScript沒有類似機(jī)制,但我們可以實(shí)現(xiàn)類似的隔離效果。
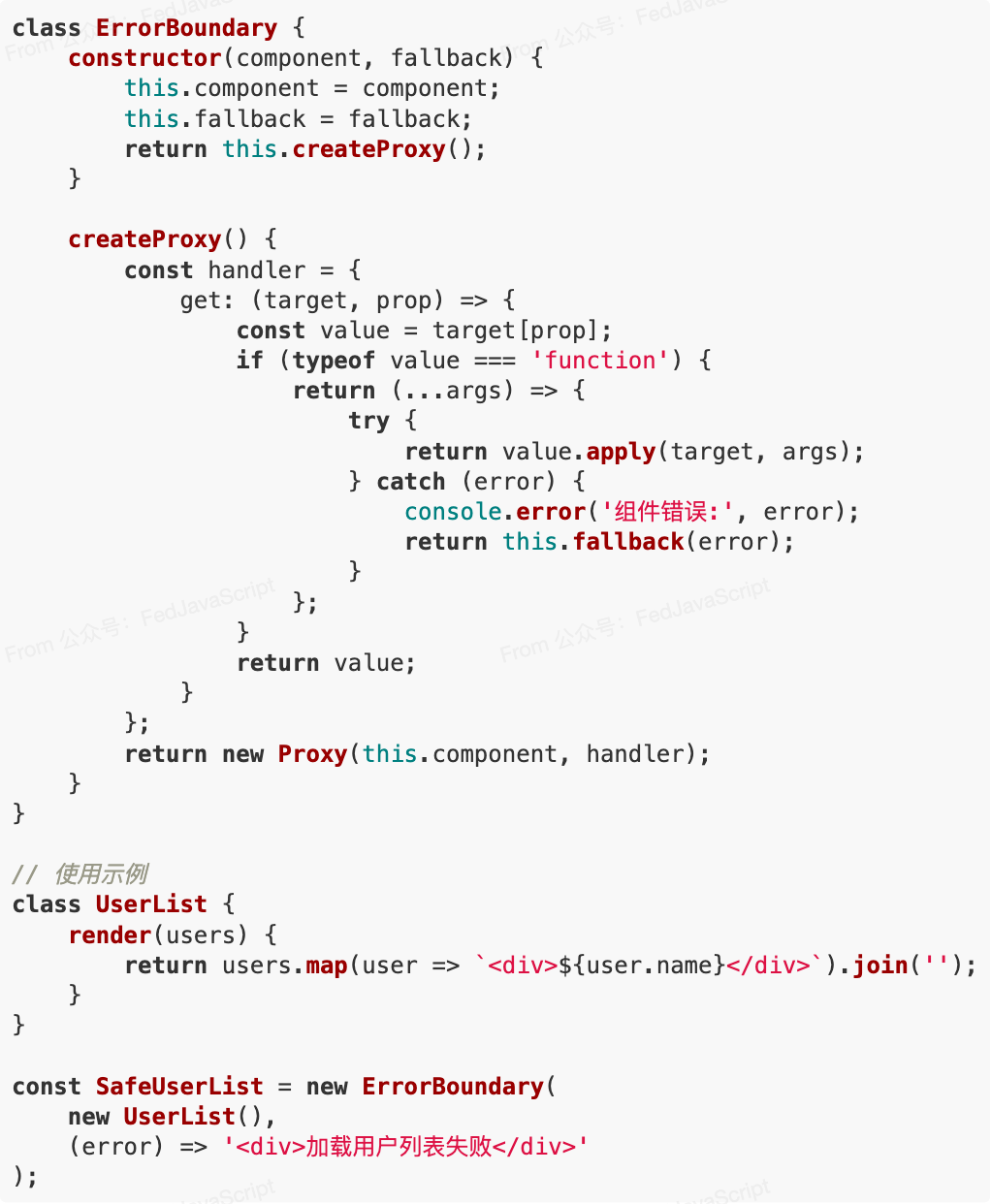
6. 優(yōu)化異步錯(cuò)誤處理鏈
使用 Promise 鏈時(shí),正確處理錯(cuò)誤傳播非常重要。
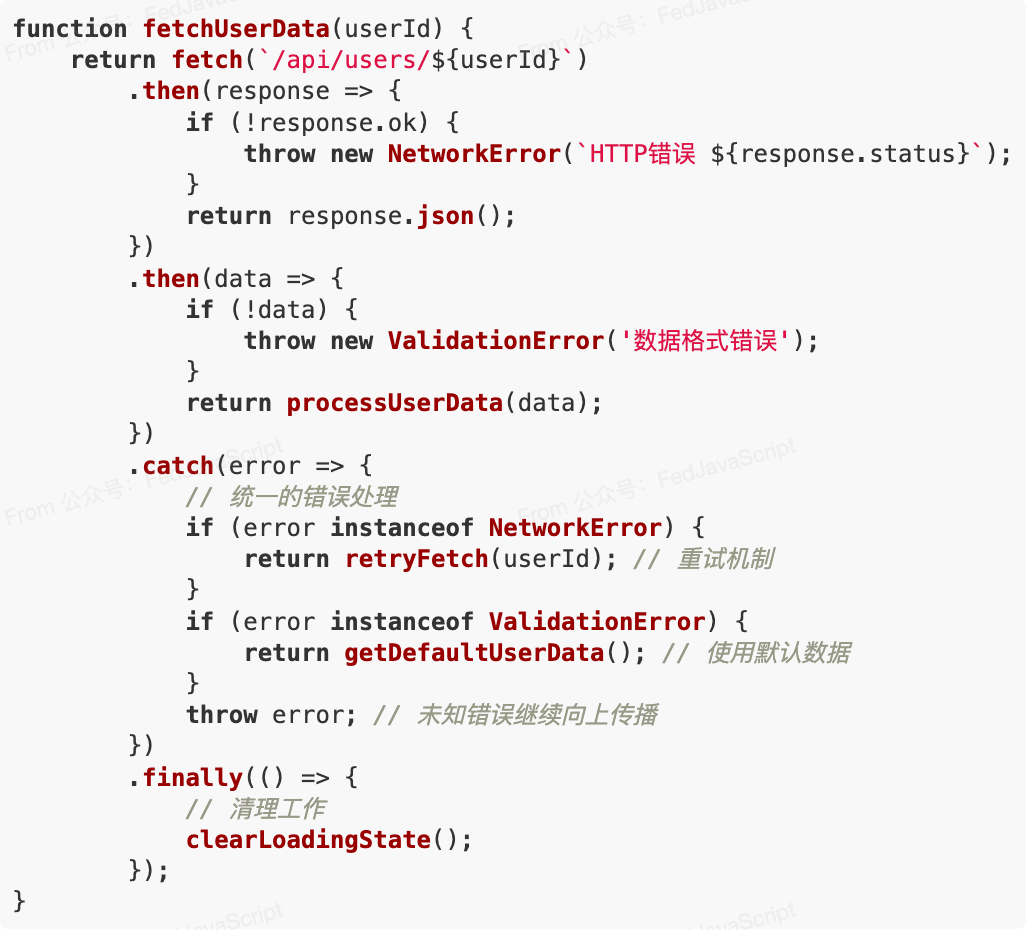
7. 日志分級(jí)處理
建立合理的日志分級(jí)系統(tǒng),可以更好地追蹤和定位問題。
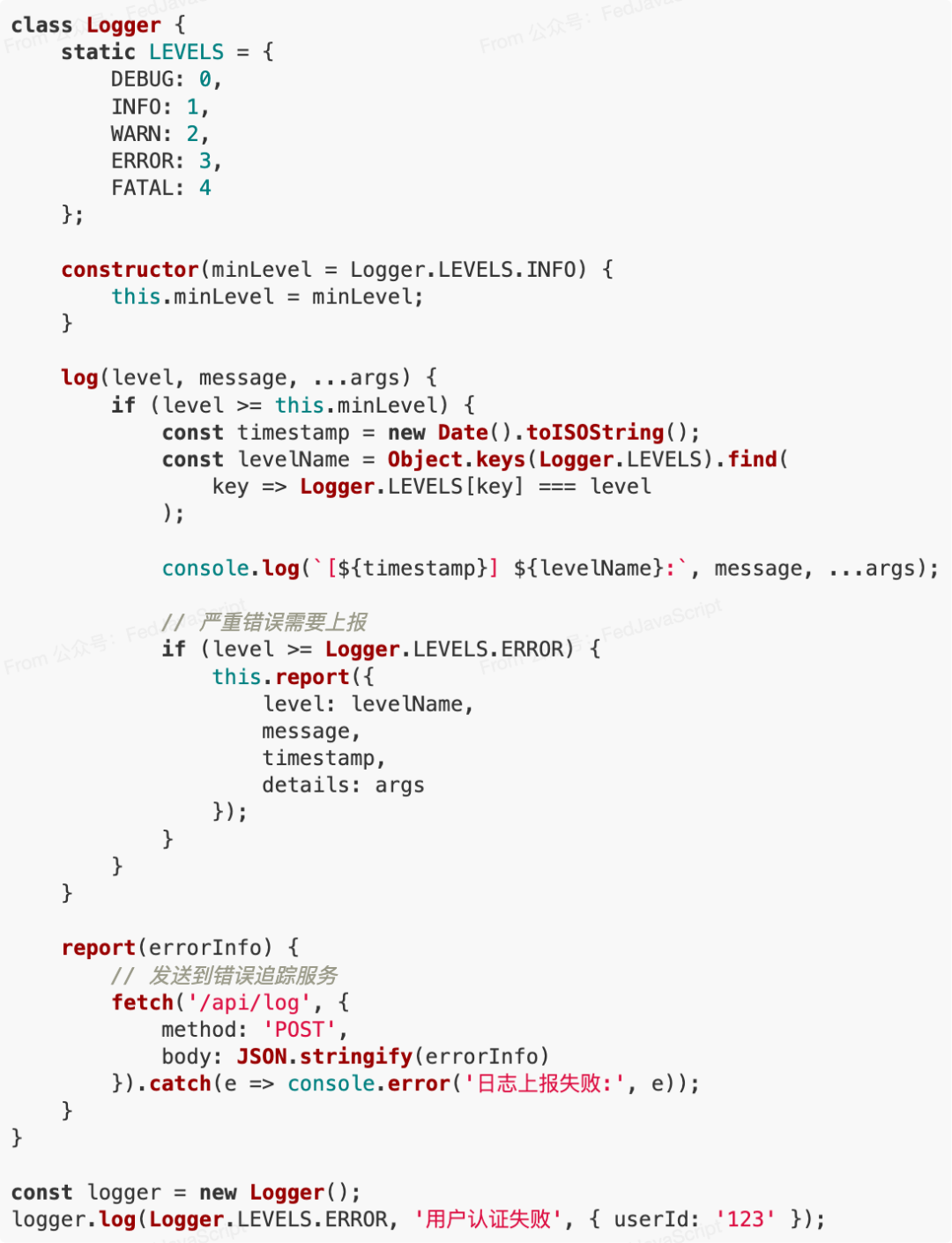
8. 錯(cuò)誤恢復(fù)機(jī)制
實(shí)現(xiàn)自動(dòng)恢復(fù)機(jī)制,讓應(yīng)用在遇到錯(cuò)誤后能夠自動(dòng)修復(fù)。
class ServiceManager {
constructor(services) {
this.services = new Map(services);
this.healthChecks = new Map();
this.startMonitoring();
}
async startService(name) {
try {
const service = this.services.get(name);
await service.start();
this.monitorService(name);
} catch (error) {
console.error(`服務(wù) ${name} 啟動(dòng)失敗:`, error);
this.attemptRecovery(name);
}
}
monitorService(name) {
const healthCheck = setInterval(async () => {
try {
const service = this.services.get(name);
const isHealthy = await service.checkHealth();
if (!isHealthy) {
throw new Error('服務(wù)健康檢查失敗');
}
} catch (error) {
this.handleServiceFailure(name, error);
}
}, 30000);
this.healthChecks.set(name, healthCheck);
}
async handleServiceFailure(name, error) {
console.error(`服務(wù) ${name} 異常:`, error);
// 停止健康檢查
clearInterval(this.healthChecks.get(name));
this.healthChecks.delete(name);
// 嘗試重啟服務(wù)
await this.attemptRecovery(name);
}
async attemptRecovery(name, retries = 3) {
for (let i = 0; i < retries; i++) {
try {
console.log(`嘗試恢復(fù)服務(wù) ${name},第 ${i + 1} 次`);
await this.startService(name);
console.log(`服務(wù) ${name} 恢復(fù)成功`);
return true;
} catch (error) {
console.error(`恢復(fù)嘗試 ${i + 1} 失敗:`, error);
await new Promise(resolve => setTimeout(resolve, 5000 * (i + 1)));
}
}
console.error(`服務(wù) ${name} 恢復(fù)失敗,已達(dá)到最大重試次數(shù)`);
return false;
}
}
好的錯(cuò)誤處理不僅僅是捕獲錯(cuò)誤,而更重要的是優(yōu)雅地處理這些錯(cuò)誤,保證應(yīng)用的正常運(yùn)行。此外,錯(cuò)誤處理應(yīng)該是一個(gè)持續(xù)改進(jìn)的過程,隨著應(yīng)用的發(fā)展,我們需要不斷完善錯(cuò)誤處理機(jī)制,以應(yīng)對(duì)新出現(xiàn)的問題和挑戰(zhàn)。
該文章在 2025/1/18 10:31:55 編輯過